switch Statement in C Language
The switch statement in C language checks the value of a variable and compares it with multiple cases. Once the case match is found, the block of statements associated with that particular case is executed. It is an alternate to else-if ladder statement. If a case match is not found, then the default case is executed. The syntax for nested if-else statement is as follows:-
switch(expression)
{
case value_1:
//code to be executed
break;
case value_2:
//code to be executed
break;
case value_3:
//code to be executed
break;
...
case value_n:
//code to be executed
break;
default:
//code to be executed if all cases are not matched
break;
}
#include<stdio.h> #include<conio.h> void main() { int no; printf("Enter A Number Between 1 To 7: "); scanf("%d",&no); switch(no) { case 1: printf("Monday"); break; case 2: printf("Tuesday"); break; case 3: printf("Wednesday"); break; case 4: printf("Thursday"); break; case 5: printf("Friday"); break; case 6: printf("Saturday"); break; case 7: printf("Sunday"); break; default: printf("Invalid Number"); } getch(); }
Flow Chart of switch Statement
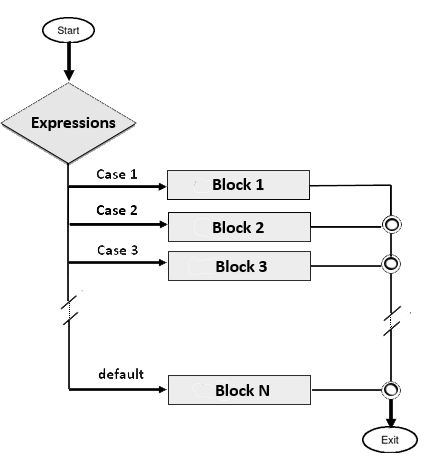